1. 개발 현황
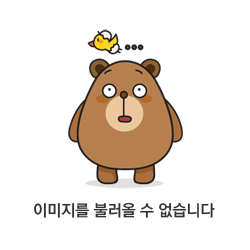
2. 상세 개발 내용
Imgui::Image를 활용하기 위해서는 D3D12_GPU_DESCRIPTOR_HANDLE를 활용해 Texture가 저장된 위치를 접근하는 방식을 활용해야 함.
3. TODO
렌더링 된 이미지를 출력하는 방식으로 기능 개선
4. 참고자료
Image Loading and Displaying Examples
Dear ImGui: Bloat-free Graphical User interface for C++ with minimal dependencies - ocornut/imgui
github.com
imgui_demo.cpp 중 Image 관련 항목
if (ImGui::TreeNode("Images"))
{
ImGuiIO& io = ImGui::GetIO();
ImGui::TextWrapped(
"Below we are displaying the font texture (which is the only texture we have access to in this demo). "
"Use the 'ImTextureID' type as storage to pass pointers or identifier to your own texture data. "
"Hover the texture for a zoomed view!");
// Below we are displaying the font texture because it is the only texture we have access to inside the demo!
// Remember that ImTextureID is just storage for whatever you want it to be. It is essentially a value that
// will be passed to the rendering backend via the ImDrawCmd structure.
// If you use one of the default imgui_impl_XXXX.cpp rendering backend, they all have comments at the top
// of their respective source file to specify what they expect to be stored in ImTextureID, for example:
// - The imgui_impl_dx11.cpp renderer expect a 'ID3D11ShaderResourceView*' pointer
// - The imgui_impl_opengl3.cpp renderer expect a GLuint OpenGL texture identifier, etc.
// More:
// - If you decided that ImTextureID = MyEngineTexture*, then you can pass your MyEngineTexture* pointers
// to ImGui::Image(), and gather width/height through your own functions, etc.
// - You can use ShowMetricsWindow() to inspect the draw data that are being passed to your renderer,
// it will help you debug issues if you are confused about it.
// - Consider using the lower-level ImDrawList::AddImage() API, via ImGui::GetWindowDrawList()->AddImage().
// - Read https://github.com/ocornut/imgui/blob/master/docs/FAQ.md
// - Read https://github.com/ocornut/imgui/wiki/Image-Loading-and-Displaying-Examples
ImTextureID my_tex_id = io.Fonts->TexID;
float my_tex_w = (float)io.Fonts->TexWidth;
float my_tex_h = (float)io.Fonts->TexHeight;
{
static bool use_text_color_for_tint = false;
ImGui::Checkbox("Use Text Color for Tint", &use_text_color_for_tint);
ImGui::Text("%.0fx%.0f", my_tex_w, my_tex_h);
ImVec2 pos = ImGui::GetCursorScreenPos();
ImVec2 uv_min = ImVec2(0.0f, 0.0f); // Top-left
ImVec2 uv_max = ImVec2(1.0f, 1.0f); // Lower-right
ImVec4 tint_col = use_text_color_for_tint ? ImGui::GetStyleColorVec4(ImGuiCol_Text) : ImVec4(1.0f, 1.0f, 1.0f, 1.0f); // No tint
ImVec4 border_col = ImGui::GetStyleColorVec4(ImGuiCol_Border);
ImGui::Image(my_tex_id, ImVec2(my_tex_w, my_tex_h), uv_min, uv_max, tint_col, border_col);
if (ImGui::BeginItemTooltip())
{
float region_sz = 32.0f;
float region_x = io.MousePos.x - pos.x - region_sz * 0.5f;
float region_y = io.MousePos.y - pos.y - region_sz * 0.5f;
float zoom = 4.0f;
if (region_x < 0.0f) { region_x = 0.0f; }
else if (region_x > my_tex_w - region_sz) { region_x = my_tex_w - region_sz; }
if (region_y < 0.0f) { region_y = 0.0f; }
else if (region_y > my_tex_h - region_sz) { region_y = my_tex_h - region_sz; }
ImGui::Text("Min: (%.2f, %.2f)", region_x, region_y);
ImGui::Text("Max: (%.2f, %.2f)", region_x + region_sz, region_y + region_sz);
ImVec2 uv0 = ImVec2((region_x) / my_tex_w, (region_y) / my_tex_h);
ImVec2 uv1 = ImVec2((region_x + region_sz) / my_tex_w, (region_y + region_sz) / my_tex_h);
ImGui::Image(my_tex_id, ImVec2(region_sz * zoom, region_sz * zoom), uv0, uv1, tint_col, border_col);
ImGui::EndTooltip();
}
}
IMGUI_DEMO_MARKER("Widgets/Images/Textured buttons");
ImGui::TextWrapped("And now some textured buttons..");
static int pressed_count = 0;
for (int i = 0; i < 8; i++)
{
// UV coordinates are often (0.0f, 0.0f) and (1.0f, 1.0f) to display an entire textures.
// Here are trying to display only a 32x32 pixels area of the texture, hence the UV computation.
// Read about UV coordinates here: https://github.com/ocornut/imgui/wiki/Image-Loading-and-Displaying-Examples
ImGui::PushID(i);
if (i > 0)
ImGui::PushStyleVar(ImGuiStyleVar_FramePadding, ImVec2(i - 1.0f, i - 1.0f));
ImVec2 size = ImVec2(32.0f, 32.0f); // Size of the image we want to make visible
ImVec2 uv0 = ImVec2(0.0f, 0.0f); // UV coordinates for lower-left
ImVec2 uv1 = ImVec2(32.0f / my_tex_w, 32.0f / my_tex_h); // UV coordinates for (32,32) in our texture
ImVec4 bg_col = ImVec4(0.0f, 0.0f, 0.0f, 1.0f); // Black background
ImVec4 tint_col = ImVec4(1.0f, 1.0f, 1.0f, 1.0f); // No tint
if (ImGui::ImageButton("", my_tex_id, size, uv0, uv1, bg_col, tint_col))
pressed_count += 1;
if (i > 0)
ImGui::PopStyleVar();
ImGui::PopID();
ImGui::SameLine();
}
ImGui::NewLine();
ImGui::Text("Pressed %d times.", pressed_count);
ImGui::TreePop();
}
728x90
반응형
'---------개인공부-------- > |DirectX (C++)|' 카테고리의 다른 글
[DX12] Frame Generation 구현 - Day 1. Optical Flow SDK 활용 환경 구성 (0) | 2025.03.23 |
---|---|
[DirectX12] Instancing 구현 (0) | 2025.02.10 |
[DirectX12] Geometry Shader 응용 (0) | 2025.01.25 |
[DirectX12] Imgui Render Target 출력 (0) | 2025.01.22 |
[DirectX] 3D 엔진 개발 현황 (0) | 2025.01.20 |